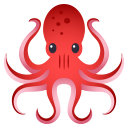
ESLint plugin to follow best practices and anticipate common mistakes when writing tests with Testing Library
You'll first need to install ESLint:
$ npm i eslint --save-dev
Next, install eslint-plugin-testing-library
:
$ npm install eslint-plugin-testing-library --save-dev
Note: If you installed ESLint globally (using the -g
flag) then you must also install eslint-plugin-testing-library
globally.
Add testing-library
to the plugins section of your .eslintrc
configuration file. You can omit the eslint-plugin-
prefix:
{
"plugins": ["testing-library"]
}
Then configure the rules you want to use under the rules section.
{
"rules": {
"testing-library/await-async-query": "error",
"testing-library/no-await-sync-query": "error",
"testing-library/no-debug": "warn"
}
}
This plugin exports a recommended configuration that enforces good
Testing Library practices (you can find more info about enabled rules in
the Supported Rules section within the Configurations
column).
To enable this configuration use the extends
property in your
.eslintrc
config file:
{
"extends": ["plugin:testing-library/recommended"]
}
Starting from the premise that
DOM Testing Library
is the base for the rest of Testing Library frameworks wrappers, this
plugin also exports different configuration for those frameworks that
enforces good practices for specific rules that only apply to them (you
can find more info about enabled rules in
the Supported Rules section within the Configurations
column).
Note that frameworks configurations enable their specific rules + recommended rules.
Available frameworks configurations are:
To enable this configuration use the extends
property in your
.eslintrc
config file:
{
"extends": ["plugin:testing-library/angular"]
}
To enable this configuration use the extends
property in your
.eslintrc
config file:
{
"extends": ["plugin:testing-library/react"]
}
To enable this configuration use the extends
property in your
.eslintrc
config file:
{
"extends": ["plugin:testing-library/vue"]
}
Rule | Description | Configurations | Fixable |
---|---|---|---|
await-async-query | Enforce async queries to have proper await |
||
await-async-utils | Enforce async utils to be awaited properly | ||
await-fire-event | Enforce async fire event methods to be awaited | ||
consistent-data-testid | Ensure data-testid values match a provided regex. |
||
no-await-sync-query | Disallow unnecessary await for sync queries |
||
no-debug | Disallow the use of debug |
||
no-dom-import | Disallow importing from DOM Testing Library | ||
no-manual-cleanup | Disallow the use of cleanup |
||
no-render-in-setup | Disallow the use of render in setup functions |
||
no-wait-for-empty-callback | Disallow empty callbacks for waitFor and waitForElementToBeRemoved |
||
no-wait-for-snapshot | Ensures no snapshot is generated inside of a waitFor call |
||
prefer-explicit-assert | Suggest using explicit assertions rather than just getBy* queries |
||
prefer-find-by | Suggest using findBy* methods instead of the waitFor + getBy queries |
||
prefer-presence-queries | Enforce specific queries when checking element is present or not | ||
prefer-screen-queries | Suggest using screen while using queries | ||
prefer-wait-for | Use waitFor instead of deprecated wait methods |
Thanks goes to these wonderful people (emoji key):
This project follows the all-contributors specification. Contributions of any kind welcome!