diff --git a/example/example.js b/example/example.js
index ec0f6f1..3b8becc 100644
--- a/example/example.js
+++ b/example/example.js
@@ -3,10 +3,10 @@ var button = document.querySelector('button')
var message = document.querySelector('#message')
var repo = document.querySelector('#repo')
-document.body.appendChild(notifications.element())
+document.body.appendChild(notifications.render([]))
button.onclick = function () {
var type = document.querySelector('input:checked').value
- notifications.options.repo = repo.value
+ notifications.repo = repo.value
notifications.add({message: message.value, type: type})
}
diff --git a/index.js b/index.js
index 661136b..08d155d 100644
--- a/index.js
+++ b/index.js
@@ -1,8 +1,8 @@
-var main = require('./main')
+var Notifications = require('./main')
var style = require('./style')
var insertCss = require('insert-css')
module.exports = function (opts) {
insertCss(style)
- return main(opts)
+ return new Notifications(opts)
}
diff --git a/main.js b/main.js
index 1d1218b..f60dfbe 100644
--- a/main.js
+++ b/main.js
@@ -1,104 +1,91 @@
-var yo = require('yo-yo')
-var defaults = require('lodash.defaults')
+var Nanocomponent = require('nanocomponent')
+var html = require('nanohtml')
-module.exports = function (opts) {
- opts = opts || {}
+class Notifications extends Nanocomponent {
+ constructor (opts) {
+ opts = opts || {}
+ super()
- opts.icons = defaults(opts.icons, {
- error: 'octicon octicon-flame',
- warning: 'octicon octicon-alert',
- info: 'octicon octicon-info',
- success: 'octicon octicon-check',
- close: 'octicon octicon-x'
- })
+ this.repo = opts.repo
+ this.icons = Object.assign({
+ error: 'octicon octicon-flame',
+ warning: 'octicon octicon-alert',
+ info: 'octicon octicon-info',
+ success: 'octicon octicon-check',
+ close: 'octicon octicon-x'
+ }, opts.icons)
- var notifications = []
- var tree
-
- return {
- element: element,
- render: render,
- add: add,
- error: error,
- info: info,
- warning: warning,
- success: success,
- state: notifications,
- options: opts
- }
-
- function add (notification) {
- if (typeof notification === 'string') notification = {message: notification}
- notifications.push(notification)
- update()
- }
-
- function error (message) {
- add({type: 'error', message: message})
- }
-
- function info (message) {
- add({type: 'info', message: message})
+ this.getMessageBody = this.getMessageBody.bind(this)
+ this.notifications = []
}
- function warning (message) {
- add({type: 'warning', message: message})
+ add (notification) {
+ this.notifications.push(notification)
+ this.rerender()
}
- function success (message) {
- add({type: 'success', message: message})
+ info (text) {
+ this.add({type: 'info', message: text})
}
- function element () {
- tree = render()
- return tree
+ error (text) {
+ this.add({type: 'error', message: text})
}
- function update () {
- yo.update(tree, render())
+ warning (text) {
+ this.add({type: 'warning', message: text})
}
- function render () {
- return yo`
-
- ${
- notifications
- .map(function (notification) {
- var classNames = [
- 'notification',
- notification.closed ? 'notification-hidden' : 'notification-show',
- 'notification-' + (notification.type || 'info')
- ]
- return yo`
-
-
-
-
-
-
- ${getMessageBody(notification)}
-
-
-
-
`
- })
- }
-
`
+ success (text) {
+ this.add({type: 'success', message: text})
}
- function getMessageBody (notification) {
+ getMessageBody (notification) {
if (notification.element) {
notification.element.className = 'notification-message'
return notification.element
}
- if (opts.repo && notification.type === 'error') {
- return yo`
+ if (this.repo && notification.type === 'error') {
+ return html`
`
} else {
- return yo`${notification.message}
`
+ return html`${notification.message}
`
+ }
+ }
+
+ createElement (notifications) {
+ if (notifications) this.notifications = notifications
+ return html`${
+ notifications
+ .map((notification) => {
+ var classNames = [
+ 'notification',
+ notification.closed ? 'notification-hidden' : 'notification-show',
+ 'notification-' + (notification.type || 'info')
+ ]
+ return html`
+
+
+
+
+
+
+ ${this.getMessageBody(notification)}
+
{ notification.closed = true; this.rerender() }} class="notification-close" title="Dismiss this notification">
+
+
+
`
+ })
}
+
`
+ }
+
+ update (notifications) {
+ return notifications !== this.notifications
}
}
+
+module.exports = Notifications
diff --git a/package.json b/package.json
index 82f0d32..9f9513c 100644
--- a/package.json
+++ b/package.json
@@ -12,14 +12,15 @@
"license": "ISC",
"dependencies": {
"insert-css": "2.0.0",
- "lodash.defaults": "4.2.0",
- "yo-yo": "^1.2.2"
+ "nanocomponent": "6.5.1",
+ "nanohtml": "1.2.2"
},
"devDependencies": {
"browserify": "15.2.0",
+ "cz-conventional-changelog": "^2.1.0",
"gh-pages": "1.1.0",
- "standard": "10.0.3",
- "semantic-release": "^12.2.4"
+ "semantic-release": "^12.2.4",
+ "standard": "^11.0.1"
},
"directories": {
"example": "example"
@@ -29,15 +30,20 @@
"url": "https://github.com/finnp/dom-notifications.git"
},
"keywords": [
+ "nanohtml",
"yo-yo",
"bel",
"choo",
"atom",
- "notifications",
- "tcby"
+ "notifications"
],
"bugs": {
"url": "https://github.com/finnp/dom-notifications/issues"
},
- "homepage": "https://github.com/finnp/dom-notifications#readme"
+ "homepage": "https://github.com/finnp/dom-notifications#readme",
+ "config": {
+ "commitizen": {
+ "path": "./node_modules/cz-conventional-changelog"
+ }
+ }
}
diff --git a/readme.md b/readme.md
index 3f278c7..7776c8d 100644
--- a/readme.md
+++ b/readme.md
@@ -1,7 +1,7 @@
-# dom-notifications [](https://github.com/semantic-release/semantic-release)
-
+# dom-notifications
+[](https://github.com/semantic-release/semantic-release)
[](https://greenkeeper.io/)
-[](https://github.com/feross/standard)
+[](https://github.com/choojs/nanocomponent)
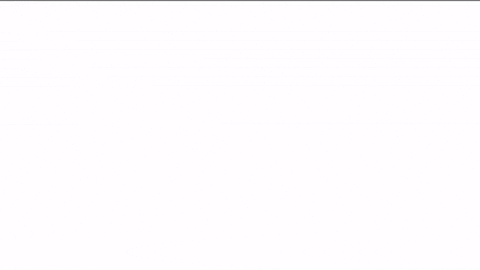
@@ -16,7 +16,7 @@ Install with `npm install dom-notifications --save` and use something like
var domNotifications = require('dom-notifications')
var notifications = domNotifications(options)
-document.body.appendChild(notifications.element())
+document.body.appendChild(notifications.render())
notifications.add({message: 'You are now logged in'}) // defaults to `info`
notifications.add({message: 'This is a warning', type: 'warning'})
@@ -51,21 +51,22 @@ button to the error notifications.
If you need more customization, instead of using the `message` property, you
can also specify an `element` property and set it to `DOMElement` that will be the content.
-For example with [yo-yo](https://github.com/maxogden/yo-yo):
+For example with [nanohtml](http://github.com/choojs/nanohtml):
```js
notifications.add({
type: 'error',
- element: yo`
+ element: html`
My super custom message!
`
})
```
+Notifications extends [Nanocomponent](https://github.com/choojs/nanocomponent).
-### `notifications.element()`
+### `notifications.render(state?)`
Creates the root element for the component. Call this ones to append it to
-the DOM.
+the DOM. Optionally state is an array of notifications
### `notifications.add(notification)`
@@ -93,7 +94,9 @@ If you don't want the styles to be used (or applied automatically),
you can also use the module like this:
```js
-var domNotifications = require('dom-notifications/main')
+var Notifications = require('dom-notifications/main')
+
+var notifications = new Notifications()
// optionally apply styles yourself
var styles = require('dom-notifications/styles')