diff --git a/articles/_raw/nextjs-vs-react-differences.md b/articles/_raw/nextjs-vs-react-differences.md new file mode 100644 index 0000000..e1fa85d --- /dev/null +++ b/articles/_raw/nextjs-vs-react-differences.md @@ -0,0 +1,221 @@ +--- +title: Next.js vs React – Differences and How to Choose the Right One for Your Project +date: 2024-12-11T14:30:03.769Z +author: Okoro Emmanuel Nzube +authorURL: https://www.freecodecamp.org/news/author/Derekvibe/ +originalURL: https://www.freecodecamp.org/news/nextjs-vs-react-differences/ +posteditor: "" +proofreader: "" +--- + +As a developer, there are many tools, languages, frameworks and open-source packages you have to learn in order to make your job easier and straightforward (though the journey isn’t a straightforward one but you will get there). + + + +Some of these tools, languages, or frameworks are used daily by community members and can undergo fundamental changes in how they are implemented or written over time. + +In this article, we will explore two popular JavaScript technologies, Next.js and React.js, by comparing their key differences, examining their strengths, and offering insights to help developers choose the best option for their projects. + +## Table of Contents + +- [Table of Contents][1] + +- [Understanding React][2] + + - [Client-side Rendering][3] + + - [Use Cases for React in Web Development][4] + +- [Exploring Next.js][5] + + - [Server-side Rendering][6] + + - [Use Cases for Next.js in web development][7] + +- [Key Difference Between Next.js and React][8] + + - [Rendering Methods: client-side vs. server-side][9] + + - [Performance Considerations][10] + + - [SEO Implications][11] + + - [Scalability and Project Complexity][12] + +- [When to Use React or Next.js][13] + + - [When to use React][14] + + - [When to Use Next.js][15] + +- [Conclusion][16] + + +## Understanding React + +We often confuse ourselves as to whether React is a JavaScript framework or not, but here is the answer to that question. React is not a JavaScript framework, it is a JavaScript library. Now, these two terminologies are often interchanged or misused, but I will explain them shortly. + +A library is a collection of already-written code that can be reused or called upon when building your own project. + +**Example:** Imagine a library where you go to study. The books are already available on the shelves – you simply pick the one you need and start reading. Similarly, in programming, a library provides ready-made tools that you can use in your project without starting from scratch. + +On the other hand, a framework is like a ready-made structure that helps you build your project. It gives you a solid foundation to work with, so you don't have to start from scratch or write repetitive code. Instead, you focus on adding your own features and logic, while the framework takes care of running things at the right time and in the right way. + +**Example:** Think of a framework like a house under construction where the walls, foundation, and roof are already built. All you need to do is decide how to design the interior—like choosing the furniture, paint, and decorations. The framework handles the heavy lifting, like ensuring the house stands strong, while you focus on making it your own. Similarly, in programming, the framework provides the structure, and you add your custom logic to complete the project. + +With that out of the way, let’s move on. + +React is one of the most popular JavaScript libraries used by developers to build fast, interactive, and reliable user interfaces. It is a declarative library that helps developers create component-based web applications. Facebook developed this library in 2011 and it has been trending since then. + +Usually, when writing JavaScript code, we create a file with the extension `js`. For example: `App.js`, `script.js`, and so on. In React we create a file with the extension `jsx`. That is: `index.jsx`, `Home.jsx`, and so on. The `jsx` is a React extension that allows you to write a JavaScript code resembling HTML. The syntax, when executed, is passed through preprocessors/transpilers which transforms the HTML-looking code into a standard JavaScript code. + +At the heart of all React applications are components. Components are chunks of user interfaces (UI) which are built independently and can be reused in different parts of your project. Different components can be built separately and later brought together to form a complex user interface (UI). + +**Note:** Every React application has at least one component, commonly referred to as the root component. This component represents the entire application. Within the root component, there are often other components, known as child components, that help structure and manage different parts of the application. + +Here is a structural representation of root and child components. + +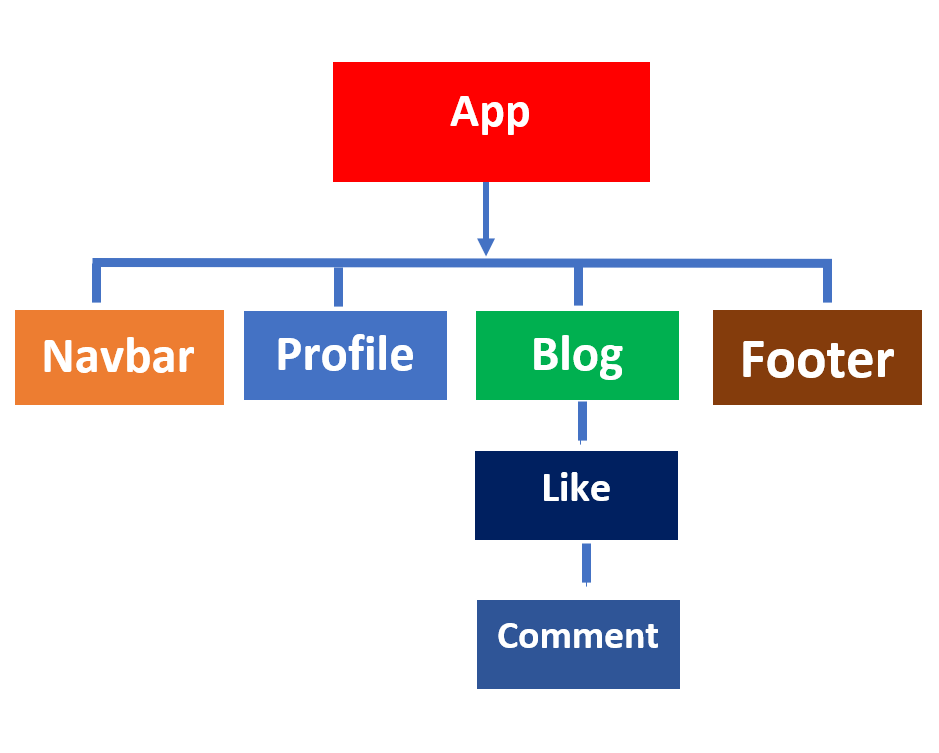 + +From the image above, you can clearly understand what components are all about. `App` is the root component, and inside the root component, we have the child components: `Navbar`, `Profile`, `Blog` and `Footer`. The child components can be reused on other pages of the project without having to rewrite the code again. + +### Client-side Rendering + +Client-side rendering (CSR) is a common technique, especially in libraries like React and frameworks like Vue.js, Angular, and so on. Here, the browser downloads and processes JavaScript files to dynamically render content directly on the user's device. With CSR, web pages are generated dynamically, and any updates or changes to the code are applied without requiring a full page reload. Only the specific parts that have changed are updated, ensuring a seamless and efficient user experience. + +Therefore, in CSR, the logic and structure of the web page is handled by the client (browser) and a fully rendered page is displayed. + +To help you understand CSR, [I have added an article here][17]. + +### **Use Cases for React in Web Development** + +Ever since React became a go-to choice for many developers, its flexibility has made it suitable for a wide range of use cases in web development. Here are a few cases: + +- **Single Page Applications (SPAs):** When we talk about SPAs, we don’t really mean that your web application has only one page, it can go on to having multiple pages. In SPAs, your web application files (HTML, CSS, JS) are generated once on your web page and when subsequent updates are made on the file, your page won’t have to fully reload. This approach helps ensure a faster transition, reduces load on the server and enhance overall user experience. + +- **Interactive User Interfaces:** React is suitable for building interactive user interfaces which, from time to time, undergo dynamic updates based on the actions of the users. Examples are online forms, dashboards, websites (E-commerce websites), and so on. + +- **Cross-Platform Applications:** Having React knowledge comes in handy when building mobile applications, simplifying the connection between web applications and mobile applications. Tools like React Native helps you achieve this process. + + +## **Exploring Next.js** + +Next.js is a popular React-based framework used in building web applications with the use of React components. Next.js provides additional structure, features, and optimization for your web application. + +Unlike React, Next.js supports server-side rendering (SSR), so requests are processed and generated from the server and then displayed on the browser (client). + +### **Server-side Rendering** + +Server-side rendering (SSR) is a technique in web development where a server generates the HTML for a web page on the server and sends it to the browser (client). In other words, the server handles the structures and logic of the page and displays a fully rendered page on the screen. + +In server-side rendering, a request is first sent to the server from the browser (client), then the server begins to process the request and when it's done processing the request, it executes the request by generating and displaying an HTML file with the content on the browser (client side). When a change is made or a new page is requested, a new request is sent again to the server and it is processed all over again – a fresh, fully rendered HTML file will be generated and displayed on the browser (client). + +For a better understanding of CSR and SSR, I have added a [YouTube video here][18]. + +### Use Cases for Next.js in web development + +- **Single Page Application (SPAs):** Next.js can be used in the creation of single page applications, similar to React. + +- **SEO-Friendly:** Next.js helps create SEO-friendly websites by rendering an HTML file on the server and delivering it to the browser. This improves search engine visibility, increasing the chances of your website appearing at the top of search results. + +- **Multi-User Platforms:** Due to Next.js ability to handle dynamic routing, API handling, and so on, it’s easy to create applications that serve various purposes. + + +## Key Difference Between Next.js and React + +### Rendering Methods: client-side vs. server-side + +When we talk about the rendering method in React, React relies mainly on rendering with the client-side rendering (CSR) method. Therefore both the logic and the structure of the web page will be handled by the browser (client). Though this method is commonly used, it has some downside effects like slower initial page load. + +Next.js, on the other hand, supports both SSR and CSR because it was built on top of React. Web pages are rendered on the server and both logic and structure of the page are all handled by the server. This enables a faster loading of the web page and also improves the SEO. + +### Performance Considerations + +In terms of performance considerations, Next.js is often preferred because it offers multiple rendering options, including server-side rendering (SSR), static site generation (SSG), Incremental Static Regeneration (ISR), and client-side rendering (CSR). In contrast, React primarily provides a single rendering approach: client-side rendering. + +### SEO Implications + +React is less SEO-friendly because search engines may struggle to index content that requires JavaScript execution to render. + +On the other hand, Next.js is more SEO-friendly than React because it renders content on the server, providing fully-rendered HTML to search engines for easier indexing. + +### Scalability and Project Complexity + +In terms of scalability and project complexity, Next.js is generally better than React. Next.js provides built-in features that enhance the scalability of your project. These include: + +- Server-side rendering (SSR) and static site generation (SSG) for better performance and SEO. + +- A built-in API routes feature for creating serverless functions seamlessly. + +- A file-based routing system that simplifies the organization of larger projects. + + +In contrast, with React, you are responsible for setting up and maintaining the structure for scalability. For larger projects, this often requires adding additional tools such as: + +- State management libraries (for example, Redux, Recoil, and so on). + +- Routing libraries (for example, React Router). + + +These tools are necessary to enhance React's scalability and address project complexity, but they also increase the overhead and effort needed to set up and manage the application. + +In summary, here is a tabular break down; + +
Factors | React | Next.js |
Scalability | It is possible but to increase scalability, it requires additional tools and a custom setup | It is scalable and already has built-in tools that increase the scalability. |
Performance | It provides only one rendering option which is client-side rendering (CSR) | It offers multiple rendering options, including SSR, SSG, ISR, and CSR. |
SEO | It is less SEO-friendly because search engines may struggle to index content that requires JavaScript execution to render. | It is more SEO-friendly than React because it renders content on the server, providing fully-rendered HTML to search engines for easier indexing. |
Use Case | Mostly used in smaller or unique projects | Mostly used in large-scale projects and enhances performance and SEO |
因素 | React | Next.js |
可扩展性 | 是可能的,但要增加可扩展性需要额外的工具和自定义设置 | 具有可扩展性且已有内置工具来提高可扩展性。 |
性能 | 仅提供一种渲染选项,即客户端渲染(CSR) | 提供多种渲染选项,包括 SSR、SSG、ISR 和 CSR。 |
SEO | SEO 友好程度较低,因为搜索引擎可能难以索引需要 JavaScript 执行才能渲染的内容。 | 比 React 更加 SEO 友好,因为它在服务器上渲染内容,向搜索引擎提供完全渲染的 HTML 以便于索引。 |
使用场景 | 主要用于较小或独特的项目 | 主要用于大规模项目,提升性能和 SEO |