-
Notifications
You must be signed in to change notification settings - Fork 492
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Merge pull request #1094 from dewanshiPaul/feature
resolved #802
- Loading branch information
Showing
2 changed files
with
77 additions
and
0 deletions.
There are no files selected for viewing
39 changes: 39 additions & 0 deletions
39
P-Problem Statements & Solution/2-Level 2/AggressiveCows.cpp
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,39 @@ | ||
#include<bits/stdc++.h> | ||
using namespace std; | ||
//function to check if cows can be allocated for the given minimum distance betwen two cows | ||
bool isAllocationPossible(vector<int>& stall, int cows, int minDist) { | ||
int cntCows = 1;//we placed one cow at the very start of the stall | ||
int recentlyPlacedPosition = stall[0]; // get the value of the start of the array as onw cow is placed | ||
|
||
//for every other stalls check | ||
for (int i = 1; i < stall.size(); i++) { | ||
//when distance between the lastly placed cow and current stall is geater than or equal to minimum distance then allocate the cow in that stall | ||
if (stall[i] - recentlyPlacedPosition >= minDist) { | ||
cntCows++; | ||
recentlyPlacedPosition = stall[i]; | ||
} | ||
} | ||
//if all cows are placed then move current allocation is possible | ||
if (cntCows == cows) return true; | ||
return false; | ||
} | ||
int main() { | ||
vector<int> stall = {1,2,4,8,9}; | ||
int cows=3; | ||
int ans; | ||
sort(stall.begin(),stall.end()); | ||
int low = 1, high = stall[stall.size() - 1] - stall[0]; | ||
//binary search starts | ||
while (low <= high) { | ||
int mid = (low + high) >> 1; | ||
if (isAllocationPossible(stall, cows, mid)) { | ||
res = mid; | ||
low = mid + 1; | ||
} else { | ||
high = mid - 1; | ||
} | ||
} | ||
// printing the answer | ||
cout << "The largest minimum distance is " << high << endl; | ||
return 0; | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,38 @@ | ||
# Aggressive Cows # | ||
|
||
## Table of Contents:- | ||
* [Problem Statement](#problem-statement) | ||
* [Explanation of Problem Statement](#explanation-of-problem-statement-with-a-test-case) | ||
* [Approach](#approach) | ||
* [Algorithm](#algorithm) | ||
* [Time Complexity](#time-complexity) | ||
|
||
## Problem Statement | ||
There is a new barn with N stalls and C number of cows. The stalls are located on a straight line with positions x1,x2,...,xN. We have to assign the cows to the stalls, is such that the minimum distance between any two of them is as large as possible. We have to find largest minimum distance. | ||
|
||
### Explanation of Problem Statement with a test case | ||
Let's say we have 5 stalls and 3 cows. The stalls are place as [1,2,4,8,9]. We will try to place 3 cows in stalls and find minimum distances from these configurations. | ||
|
||
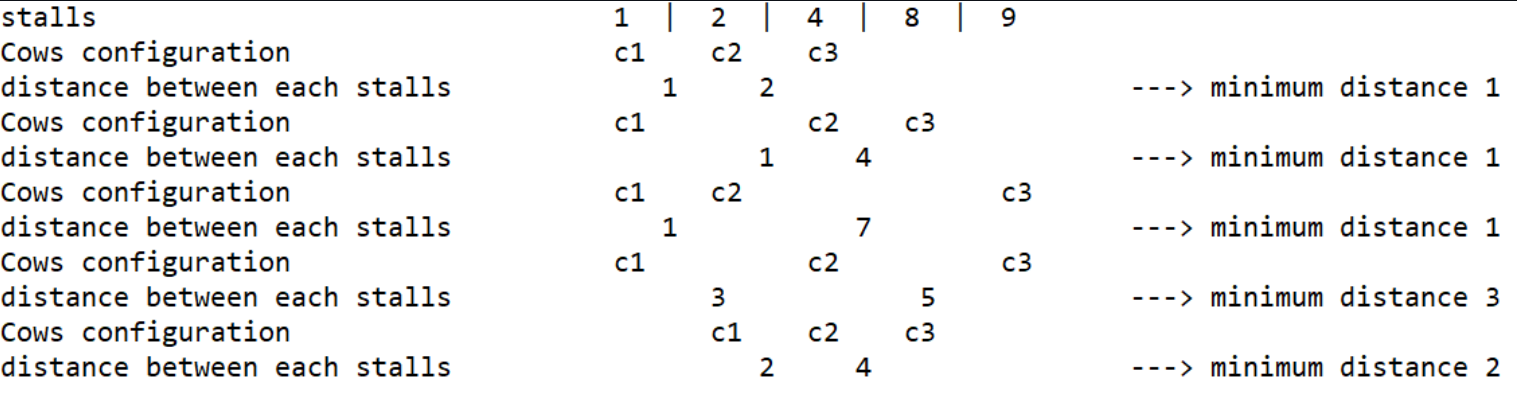 | ||
many such configurations are possible. For above shown configurations, maximum or largest minimum distance is 3. This is what we have to do. Hope you understand... else try out some more configurations on your own :) | ||
|
||
### Approach | ||
* We can try out to find all possible configurations via which cows can be placed in given number of stalls. This would be a recursive solution with exponential time complexity. Can we reduce it? | ||
* We increase the range of minimum distance between two cows and place them according. This would take n^2 solution. Umm seems better because we reduced our solution from exponential to polynomial solution. Can we improve more? | ||
* From above solution, we can see that we are alloting cows to stalls from pre-defining the minimum distance between the two cows. So, we are basically making use of range for distances. This range will be in sorted increasing order. This gives us hint to use of binary search. | ||
|
||
### Algorithm | ||
``` | ||
Sort the given array. | ||
low = 1, high = last element of the array | ||
while(low<=high): | ||
mid = (low+high)/2 | ||
for i in array: | ||
if mid is valid minimum distance for allocating cows in stalls | ||
low = mid+1 | ||
else | ||
high = mid-1 | ||
return high | ||
``` | ||
### Time Complexity | ||
For sorting, it takes Nlog(N). For our algorithm, log(N) for binary search and N for checking if cows can be allocated or not. So total time complexity is Nlog(N). |