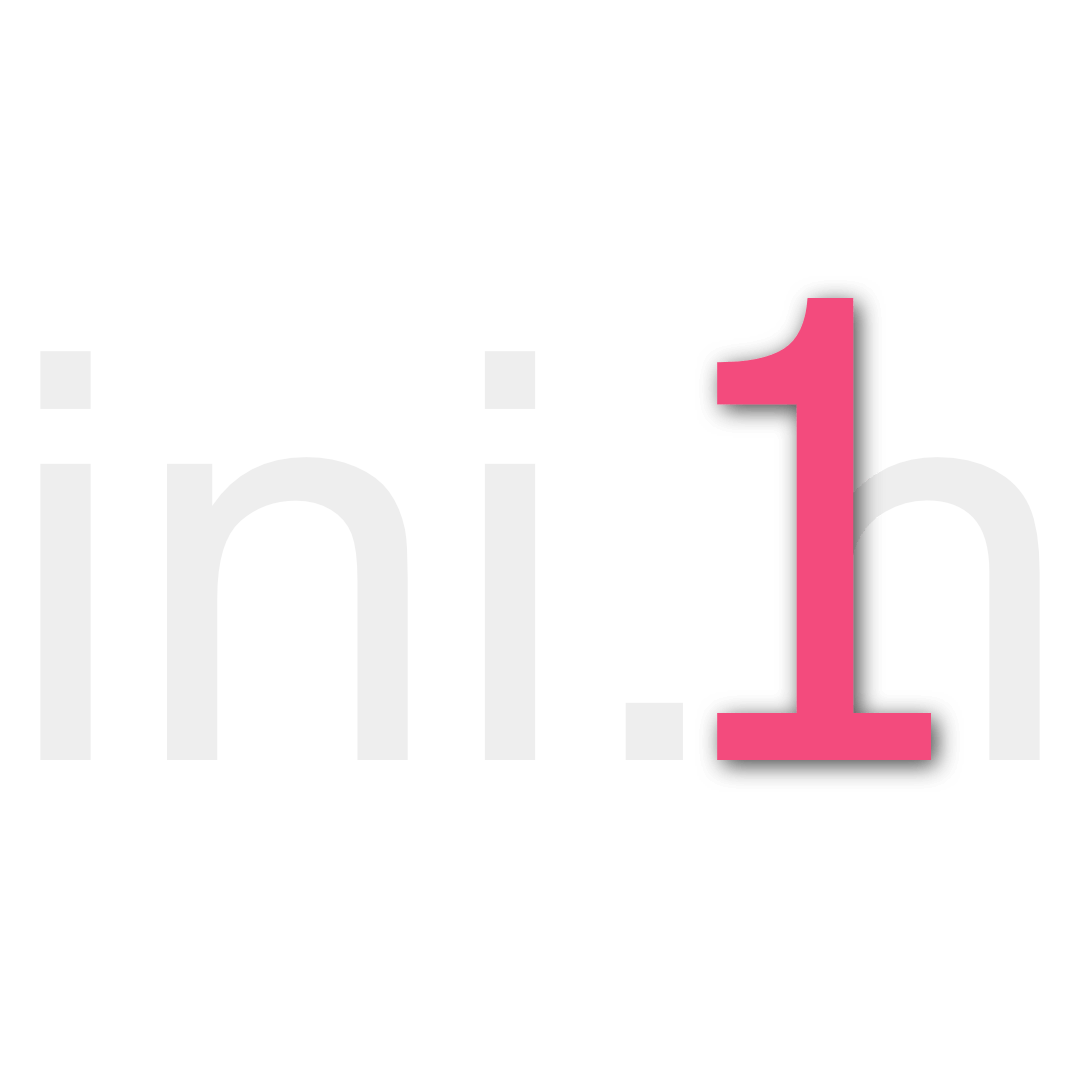
ini.h is a header-only library for reading and writing INI files in C++ through a simple, intuitive API.
To start using ini.h, copy the single header file and drop it into your project. Now you can use the library by adding the following directive:
#include "ini.h"
- C++17 or higher
There are two ways to read and parse INI files with ini.h:
- Through a file path
- Through a file stream
File Path
#include "ini.h"
int main(int argc, char* argv[])
{
ini::File file = ini::open("path/to/ini/example.ini");
}
File Stream
#include <fstream>
#include "ini.h"
int main(int argc, char* argv[])
{
std::ifstream stream("path/to/ini/example.ini");
ini::File file = ini::load(stream);
// You are responsible for closing the file stream.
stream.close();
}
As for writing, simply pass the destination path to the write
function.
Writing to an INI File
#include "ini.h"
int main(int argc, char* argv[])
{
ini::File file = ini::open("path/to/ini/example.ini");
file.add_section("New Section");
file["New Section"]["is_generic_key"] = "true";
file.write("path/to/ini/example.ini");
}
You can retrieve values by using the operator[] but this will only return a string value. If you need a value in the form of a bool, for example, you can do so by using the get
function.
Getting Values
#include "ini.h"
int main(int argc, char* argv[])
{
ini::File file = ini::open("path/to/ini/example.ini");
std::string title = file["FileInfo"]["title"];
bool auto_save_enabled = file["FileInfo"].get<bool>("autoSaveEnabled"):
size_t word_count = file["FileInfo"].get<size_t>("wordCount");
double line_spacing = file["FileInfo"].get<double>("lineSpacing");
}
example.ini
[FileInfo]
title = On the topic of C++
author = John Appleseed
lastDateOpened = 2022-07-24
autoSaveEnabled = true
wordCount = 0
lineSpacing = 1.5
Setting values with can also be done with the subscript operator but this requires a string representation of the value you're trying to set.
If you already have a bool
, a double
, etc., you can simply use the set
function. Those values will automatically be converted into a string representation for you.
Setting Values
#include "ini.h"
int main(int argc, char* argv[])
{
ini::File file = ini::open("path/to/ini/example.ini");
file["FileInfo"]["title"] = "On the topic of C#";
file["FileInfo"].set<bool>("autoSaveEnabled", false);
file["FileInfo"].set<size_t>("wordCount", 1);
file["FileInfo"].set<double>("lineSpacing", 2.0);
}
example.ini
[FileInfo]
title = On the topic of C++
author = John Appleseed
lastDateOpened = 2022-07-24
autoSaveEnabled = true
wordCount = 0
lineSpacing = 1.5
; comment text
# comment text
ℹ️ Comments must start at the beginning of a line.
Section names and keys are case-sensitive.
example.ini
[SECTION]
key = value
KEY = value
[section]
key = value
KEY = value
=
:
example.ini
[Section]
key1 = value
key2 : value
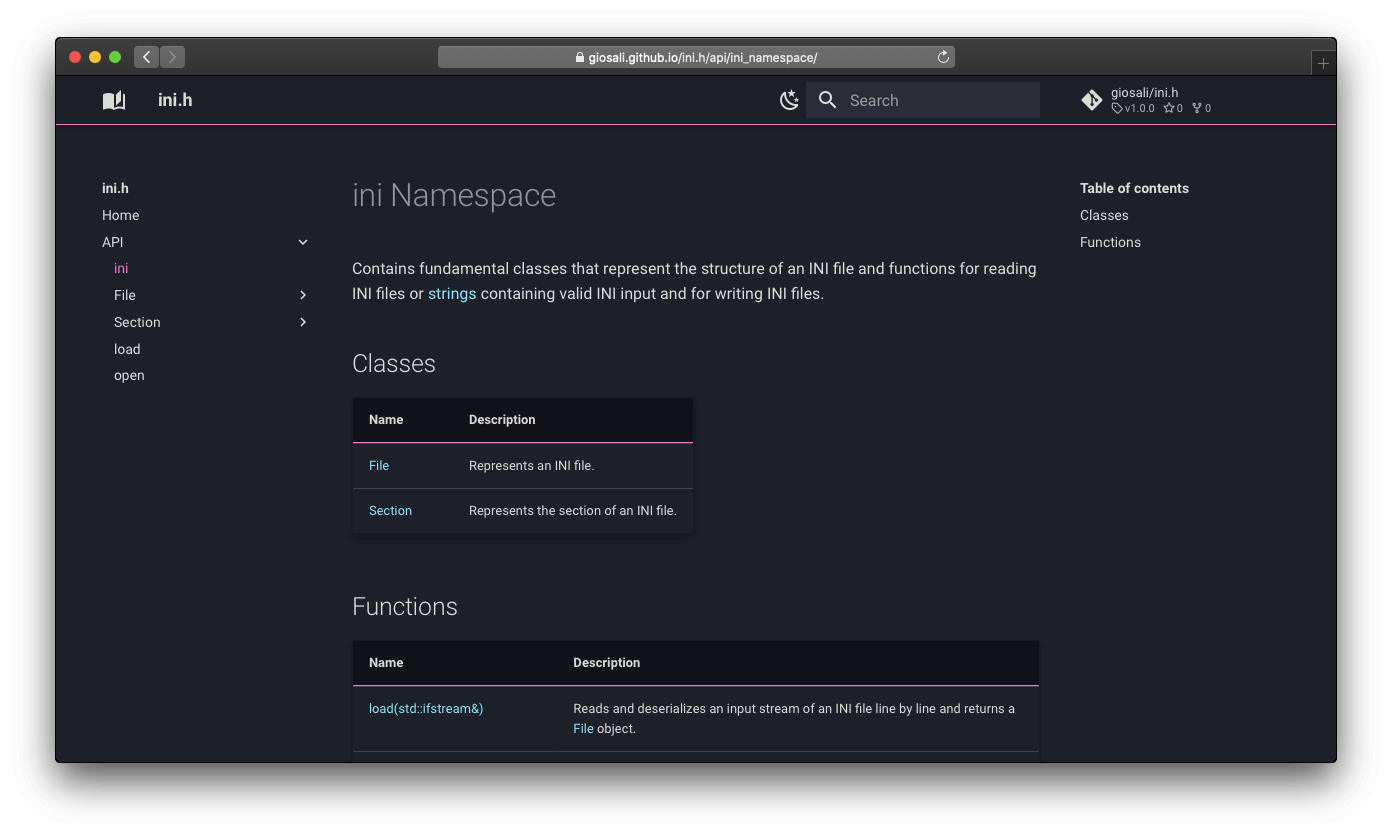
You can view more in-depth, MSDN-style documentation on the ini.h library here.