-
Notifications
You must be signed in to change notification settings - Fork 17
In app notifications
In-app messages are a type of communication tool with mobile users that includes banners, pop-ups and other formats that appear on screen of mobile applications while users are interacting with it. Read more documentation
Feature is available since 9.0.0 version, Huawei SDK 6.0.0
Full-featured In-App notifications are disabled by default. To enable them, you need to call .withFullFeaturedInApps()
. Without calling this method, Full-featured In-App notifications will trigger the MESSAGE_RECEIVED broadcast event but will not be displayed within the WebView.
Enabling this feature also enables JavaScript execution on the webView for the feature proper work
MobileMessaging.Builder(application)
.withFullFeaturedInApps()
.build()
expand to see Java code
new MobileMessaging.Builder(application)
.withFullFeaturedInApps()
.build();
After Mobile Messaging SDK is integrated into your app, you just need to create a Flow in Infobip Customer Portal, choose "Send Push or In-App notification" element and select "In-App notification", In-app notification Editor will be opened, where you can create In-app notification according to your preferences.
Banner | Pop-up | Fullscreen |
![]() |
![]() |
![]() |
Following on-tap actions are supported for the Banner and for the action buttons of other In-App notification types:
- Open URL in browser
- Open URL within the webView
- Open page in mobile app
More information can be found on the How to define specific action on notification or in-app primary button tap(open url, deeplink)?
Additionally, MobileMessaging SDK will trigger:
- notificationTapped event if user tapped the banner In-App notification
- notificationActionTapped event if user tapped an action button of the Pop-up or Fullscreen notification
-
If you do not enable the feature or use older versions of the Mobile Messaging SDK without this feature support, the messages will be received, but will not be displayed. The Broadcast event MESSAGE_RECEIVED will be triggered.
-
For Full-featured In-App messages, the
Message
object in the fieldbody
contains text "In-App" for technical reasons, but this field won't be involved in the process of displaying an In-App notification in the WebView. -
For Full-featured In-App messages, the
Message
object in the fieldsilent
will always be set to "true".
In-app notifications are alerts shown in foreground when user opens the app. Modal supported since 1.13.0. Banner supported since 2.0.0, Huawei SDK 1.0.0.
Default displaying of in-app notifications is available by using of our SDK and sending an in-app message. If you want to opt out and create your own in-app notifications use this setting.
After you integrated Mobile Messaging SDK into your app, you just need to send a message using Push HTTP single, multiple or OMNI API or Portal providing the desired inAppStyle
notification option - either MODAL
or BANNER
. The Mobile Messaging SDK will automatically display the message with appropriate style.
When inAppStyle
notification option is set to MODAL
for API call or in Portal campaign settings, the in-app notification will be shown up in the following cases:
- notification is received and application is opened by tapping on the apps icon;
- notification is received when application is in foreground state;
- for interactive notifications, user opens the app by tapping on the notification (but not performing a notification action).
Modal In-app notification is supported for silent push notifications as well as for cases when the end user opted out from push notifications at OS level, so that mobile users can be targeted with in-app message waiting them in the application and not get disturbed with notification on lock screen.
Modal In-app notification displays only the most recently received message with in-app enabled. In case of non-interactive messages, modal in-app alert will have default actions: "Cancel" and "Open".
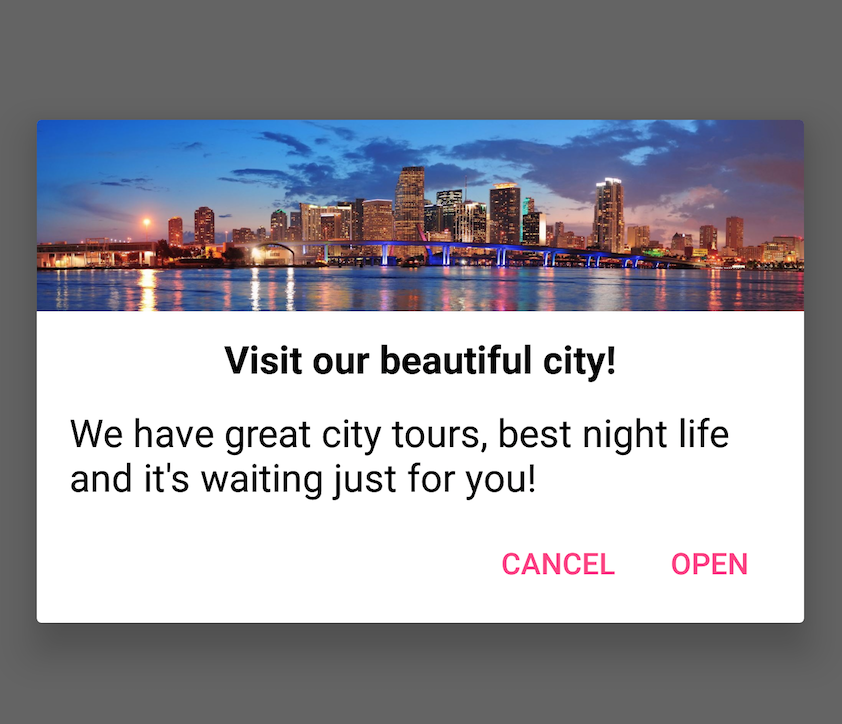
For interactive notifications, actions defined for category will be displayed.
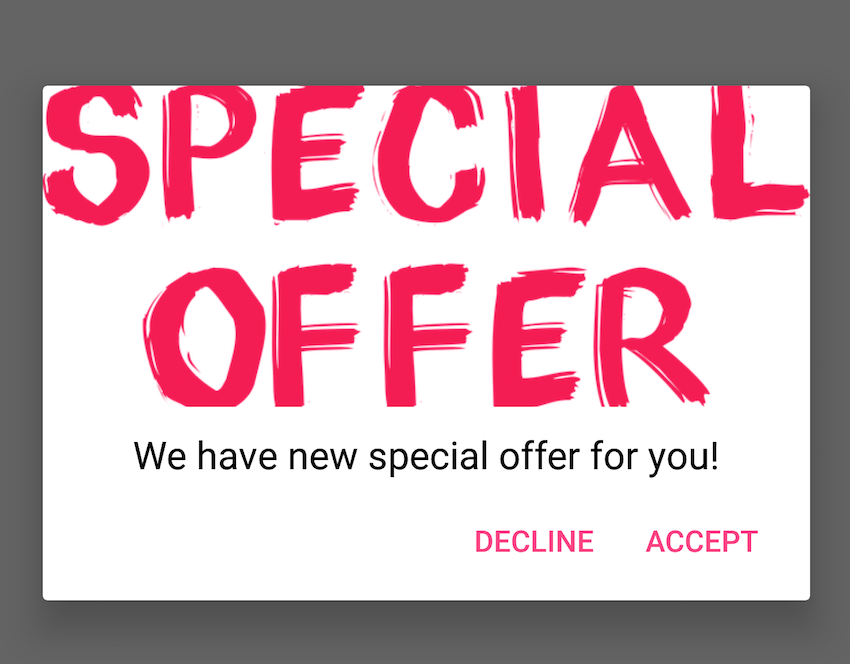
Button text color on in-app dialog is managed by style attribute colorAccent
, such as:
<style name="AppTheme" parent="Theme.AppCompat.Light.DarkActionBar">
<item name="colorAccent">@color/colorAccent</item>
</style>
Subscribe to NOTIFICATION_ACTION_TAPPED event and you're all set up to test pressed actions. If the sent notification didn’t have any category, in-app alert will be shown with default actions (localized texts):
Action | Action ID | Foreground |
---|---|---|
Cancel |
mm_cancel |
false |
Open |
mm_open |
true |
As already mentioned, for interactive notifications actions defined for category will be used.
In the event, you've subscribed to, you can check which action has been chosen and act upon it:
private val actionTappedMessageReceiver = object: BroadcastReceiver() {
override fun onReceive(context:Context, intent: Intent) {
val message = Message.createFrom(intent.extras)
val action = NotificationAction.createFrom(intent.extras)
if (action?.id == "mm_open") {
// TODO my business logic
}
}
}
override fun onResume() {
super.onResume()
// subscribe to NOTIFICATION_ACTION_TAPPED event
val localBroadcastManager = LocalBroadcastManager.getInstance(this)
localBroadcastManager.registerReceiver(actionTappedMessageReceiver, IntentFilter(InteractiveEvent.NOTIFICATION_ACTION_TAPPED.key))
}
expand to see Java code
// declare action tapped message receiver
private final BroadcastReceiver actionTappedMessageReceiver = new BroadcastReceiver() {
@Override
public void onReceive(Context context, Intent intent) {
Message message = Message.createFrom(intent.getExtras());
NotificationAction action = NotificationAction.createFrom(intent.getExtras());
if (action != null && "mm_open".equals(action.getId()) {
// TODO my business logic
}
}
};
@Override
protected void onResume() {
super.onResume();
// subscribe to NOTIFICATION_ACTION_TAPPED event
LocalBroadcastManager localBroadcastManager = LocalBroadcastManager.getInstance(this);
localBroadcastManager.registerReceiver(actionTappedMessageReceiver, new IntentFilter(InteractiveEvent.NOTIFICATION_ACTION_TAPPED.getKey()));
}
Temporary banners will simply appear at the top of your screen and then disappear after few seconds. When inAppStyle
notification option is set to BANNER
for API call or in Portal campaign settings, the in-app notification will be shown up in the following cases:
- notification is received when application is in foreground state.

It's possible to disable the Basic Modal In-App and Full-Featured In-Apps, for further details check this page - Disable In-App notifications.
If you have any questions or suggestions, feel free to send an email to support@infobip.com or create an issue.
- Library events
- Server errors
- User profile
- Messages and notifications management
- Inbox
- Geofencing API
- Android Manifest components
- Privacy settings
- In-app chat
- Infobip RTC calls and UI
- Backup rules