-
Notifications
You must be signed in to change notification settings - Fork 25
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
feat: initial implementation #1
Changes from all commits
File filter
Filter by extension
Conversations
Jump to
Diff view
Diff view
There are no files selected for viewing
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,64 @@ | ||
dist/ | ||
|
||
# Logs | ||
logs | ||
*.log | ||
npm-debug.log* | ||
yarn-debug.log* | ||
yarn-error.log* | ||
|
||
# Runtime data | ||
pids | ||
*.pid | ||
*.seed | ||
*.pid.lock | ||
|
||
# Directory for instrumented libs generated by jscoverage/JSCover | ||
lib-cov | ||
|
||
# Coverage directory used by tools like istanbul | ||
coverage | ||
|
||
# nyc test coverage | ||
.nyc_output | ||
|
||
# Grunt intermediate storage (http://gruntjs.com/creating-plugins#storing-task-files) | ||
.grunt | ||
|
||
# Bower dependency directory (https://bower.io/) | ||
bower_components | ||
|
||
# node-waf configuration | ||
.lock-wscript | ||
|
||
# Compiled binary addons (http://nodejs.org/api/addons.html) | ||
build/Release | ||
|
||
# Dependency directories | ||
node_modules/ | ||
jspm_packages/ | ||
|
||
# Typescript v1 declaration files | ||
typings/ | ||
|
||
# Optional npm cache directory | ||
.npm | ||
|
||
# Optional eslint cache | ||
.eslintcache | ||
|
||
# Optional REPL history | ||
.node_repl_history | ||
|
||
# Output of 'npm pack' | ||
*.tgz | ||
|
||
# Yarn Integrity file | ||
.yarn-integrity | ||
|
||
# dotenv environment variables file | ||
.env | ||
|
||
# while testing npm5 | ||
package-lock.json | ||
yarn.lock |
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,188 @@ | ||
# IPNS | ||
|
||
[](http://protocol.ai) | ||
[](http://ipfs.io/) | ||
[](http://webchat.freenode.net/?channels=%23ipfs) | ||
[](https://github.com/RichardLitt/standard-readme) | ||
[](https://github.com/feross/standard) | ||
|
||
> ipns record definitions | ||
|
||
This module contains all the necessary code for creating, understanding and validating IPNS records. | ||
|
||
## Lead Maintainer | ||
|
||
[Vasco Santos](https://github.com/vasco-santos). | ||
|
||
## Table of Contents | ||
|
||
- [Install](#install) | ||
- [Usage](#usage) | ||
- [Create Record](#create-record) | ||
- [Validate Record](#validate-record) | ||
- [Embed public key to record](#embed-public-key-to-record) | ||
- [Extract public key from record](#extract-public-key-from-record) | ||
- [Datastore key](#datastore-key) | ||
- [API](#api) | ||
- [Contribute](#contribute) | ||
- [License](#license) | ||
|
||
### Install | ||
|
||
> npm install ipns | ||
|
||
## Usage | ||
|
||
#### Create record | ||
|
||
```js | ||
const ipns = require('ipns') | ||
|
||
ipns.create(privateKey, value, sequenceNumber, lifetime, (err, entryData) => { | ||
// your code goes here | ||
}); | ||
``` | ||
|
||
#### Validate record | ||
|
||
```js | ||
const ipns = require('ipns') | ||
|
||
ipns.validate(publicKey, ipnsEntry, (err) => { | ||
// your code goes here | ||
// if no error, the record is valid | ||
}); | ||
``` | ||
|
||
#### Embed public key to record | ||
|
||
> Not available yet | ||
|
||
#### Extract public key from record | ||
|
||
> Not available yet | ||
|
||
#### Datastore key | ||
|
||
```js | ||
const ipns = require('ipns') | ||
|
||
ipns.getLocalKey(peerId); | ||
``` | ||
|
||
Returns a key to be used for storing the ipns entry locally, that is: | ||
|
||
``` | ||
/ipns/${base32(<HASH>)} | ||
``` | ||
|
||
#### Marshal data with proto buffer | ||
|
||
```js | ||
const ipns = require('ipns') | ||
|
||
ipns.create(privateKey, value, sequenceNumber, lifetime, (err, entryData) => { | ||
// ... | ||
const marshalledData = ipns.marshal(entryData) | ||
// ... | ||
}); | ||
``` | ||
|
||
Returns the entry data serialized. | ||
|
||
#### Unmarshal data from proto buffer | ||
|
||
```js | ||
const ipns = require('ipns') | ||
|
||
const data = ipns.unmarshal(storedData) | ||
``` | ||
|
||
Returns the entry data structure after being serialized. | ||
|
||
## API | ||
|
||
#### Create record | ||
|
||
```js | ||
|
||
ipns.create(privateKey, value, sequenceNumber, lifetime, [callback]); | ||
``` | ||
|
||
Create an IPNS record for being stored in a protocol buffer. | ||
|
||
- `privateKey` (`PrivKey` [RSA Instance](https://github.com/libp2p/js-libp2p-crypto/blob/master/src/keys/rsa-class.js)): key to be used for cryptographic operations. | ||
- `value` (string): ipfs path of the object to be published. | ||
- `sequenceNumber` (Number): number representing the current version of the record. | ||
- `lifetime` (string): lifetime of the record (in milliseconds). | ||
- `callback` (function): operation result. | ||
|
||
`callback` must follow `function (err, ipnsEntry) {}` signature, where `err` is an error if the operation was not successful. `ipnsEntry` is an object that contains the entry's properties, such as: | ||
|
||
```js | ||
{ | ||
value: '/ipfs/QmWEekX7EZLUd9VXRNMRXW3LXe4F6x7mB8oPxY5XLptrBq', | ||
signature: Buffer, | ||
validityType: 0, | ||
validity: '2018-06-27T14:49:14.074000000Z', | ||
sequence: 2 | ||
} | ||
``` | ||
|
||
#### Validate record | ||
|
||
```js | ||
|
||
ipns.validate(publicKey, ipnsEntry, [callback]); | ||
``` | ||
|
||
Validate an IPNS record previously stored in a protocol buffer. | ||
|
||
- `publicKey` (`PubKey` [RSA Instance](https://github.com/libp2p/js-libp2p-crypto/blob/master/src/keys/rsa-class.js)): key to be used for cryptographic operations. | ||
- `ipnsEntry` (Object): ipns entry record (obtained using the create function). | ||
- `callback` (function): operation result. | ||
|
||
`callback` must follow `function (err) {}` signature, where `err` is an error if the operation was not successful. This way, if no error, the validation was successful. | ||
|
||
#### Datastore key | ||
|
||
```js | ||
ipns.getDatastoreKey(peerId); | ||
``` | ||
|
||
Get a key for storing the ipns entry in the datastore. | ||
|
||
- `peerId` (`Uint8Array`): peer identifier. | ||
|
||
#### Marshal data with proto buffer | ||
|
||
```js | ||
const marshalledData = ipns.marshal(entryData) | ||
}); | ||
``` | ||
|
||
Returns the entry data serialized. | ||
|
||
- `entryData` (Object): ipns entry record (obtained using the create function). | ||
|
||
#### Unmarshal data from proto buffer | ||
|
||
```js | ||
const data = ipns.unmarshal(storedData) | ||
``` | ||
|
||
Returns the entry data structure after being serialized. | ||
|
||
- `storedData` (Buffer): ipns entry record serialized. | ||
|
||
## Contribute | ||
|
||
Feel free to join in. All welcome. Open an [issue](https://github.com/ipfs/js-ipns/issues)! | ||
|
||
This repository falls under the IPFS [Code of Conduct](https://github.com/ipfs/community/blob/master/code-of-conduct.md). | ||
|
||
[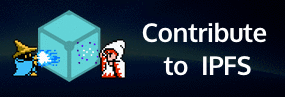](https://github.com/ipfs/community/blob/master/contributing.md) | ||
|
||
## License | ||
|
||
Copyright (c) Protocol Labs, Inc. under the **MIT**. See [MIT](./LICENSE) for details. |
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,2 @@ | ||
// Warning: This file is automatically synced from https://github.com/ipfs/ci-sync so if you want to change it, please change it there and ask someone to sync all repositories. | ||
javascript() |
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,57 @@ | ||
{ | ||
"name": "ipns", | ||
"version": "0.1.0", | ||
"description": "ipns record definitions", | ||
There was a problem hiding this comment. Choose a reason for hiding this commentThe reason will be displayed to describe this comment to others. Learn more. Is there going to more than just record stuff in here? If not would it be better named There was a problem hiding this comment. Choose a reason for hiding this commentThe reason will be displayed to describe this comment to others. Learn more. I totally agree with you! It has been discussed here, but there is no agreement yet! It is |
||
"leadMaintainer": "Vasco Santos <vasco.santos@moxy.studio>", | ||
"main": "src/index.js", | ||
"scripts": { | ||
"build": "aegir build", | ||
"lint": "aegir lint", | ||
"release": "aegir release", | ||
"release-minor": "aegir release --type minor", | ||
"release-major": "aegir release --type major", | ||
"test": "aegir test", | ||
"test:browser": "aegir test -t browser -t webworker", | ||
"test:node": "aegir test -t node" | ||
}, | ||
"pre-push": [ | ||
"lint", | ||
"test" | ||
], | ||
"repository": { | ||
"type": "git", | ||
"url": "git+https://github.com/ipfs/js-ipns.git" | ||
}, | ||
"keywords": [ | ||
"ipfs", | ||
"ipns" | ||
], | ||
"author": "Vasco Santos <vasco.santos@moxy.studio>", | ||
"license": "MIT", | ||
"bugs": { | ||
"url": "https://github.com/ipfs/js-ipns/issues" | ||
}, | ||
"homepage": "https://github.com/ipfs/js-ipns#readme", | ||
"dependencies": { | ||
"base32-encode": "^1.1.0", | ||
"big.js": "^5.1.2", | ||
"debug": "^3.1.0", | ||
"left-pad": "^1.3.0", | ||
"nano-date": "^2.1.0", | ||
"protons": "^1.0.1" | ||
}, | ||
"devDependencies": { | ||
"aegir": "^13.1.0", | ||
"chai": "^4.1.2", | ||
"chai-bytes": "^0.1.1", | ||
"chai-string": "^1.4.0", | ||
"dirty-chai": "^2.0.1", | ||
"ipfs": "^0.29.3", | ||
"ipfsd-ctl": "^0.36.0", | ||
"libp2p-crypto": "^0.13.0", | ||
"multihashes": "^0.4.13" | ||
}, | ||
"contributors": [ | ||
"Vasco Santos <vasco.santos@moxy.studio>" | ||
] | ||
} |
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,7 @@ | ||
'use strict' | ||
|
||
exports.ERR_IPNS_EXPIRED_RECORD = 'ERR_IPNS_EXPIRED_RECORD' | ||
exports.ERR_UNRECOGNIZED_VALIDITY = 'ERR_UNRECOGNIZED_VALIDITY' | ||
exports.ERR_SIGNATURE_CREATION = 'ERR_SIGNATURE_CREATION' | ||
exports.ERR_SIGNATURE_VERIFICATION = 'ERR_SIGNATURE_VERIFICATION' | ||
exports.ERR_UNRECOGNIZED_FORMAT = 'ERR_UNRECOGNIZED_FORMAT' |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
This doesn't feel like it belongs here...shouldn't it be something that the datastore determines?
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Ok, maybe the name was not the best one. For
ipns
there are two different ways of storing records (which can be used together), store locally, and store using routing.In addition, the local store is being used with datastore now, but it should also be compatible with any other
key-value
store.Therefore, I will change this name to
getLocalKey
, which I think that suits better here. What do you think?