π Product & Resources - Here
π Help Center - Here
πΌ KYC Verification Demo - Here
πββοΈ Docker Hub - Here
This repo performs face auto-capture
functionality on real-time mobile camera.
It also supports the following functionalities: face liveness detection
, face recognition
, pose estimation
, face quality calculation
, face landmark detection
, face occlusion detection
, eye closure detection
, age/gender estimation
.
In this repository, we integrated KBY-AI's
face premium SDK
into the Flutter project for both Android and iOS.
Basic | Standard | π½ Premium |
---|---|---|
Face Detection | Face Detection | Face Detection |
Face Liveness Detection | Face Liveness Detection | Face Liveness Detection |
Pose Estimation | Pose Estimation | Pose Estimation |
Face Recognition | Face Recognition | |
68 points Face Landmark Detection | ||
Face Quality Calculation | ||
Face Occlusion Detection | ||
Eye Closure Detection | ||
Age, Gender Estimation |
No. | Repository | SDK Details |
---|---|---|
1 | Face Liveness Detection - Android | Basic SDK |
2 | Face Liveness Detection - iOS | Basic SDK |
3 | Face Recognition - Android | Standard SDK |
4 | Face Recognition - iOS | Standard SDK |
5 | Face Recognition - Flutter | Standard SDK |
6 | Face Recognition - Ionic-Cordova | Standard SDK |
7 | Face Recognition - React-Native | Standard SDK |
8 | Face Attribute - Android | Premium SDK |
9 | Face Attribute - iOS | Premium SDK |
β‘οΈ | Face Attribute - Flutter | Premium SDK |
To get Face SDK(server), please visit products here.
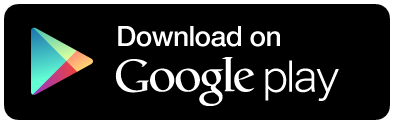
To run this repo successfully, license should be required based on each application ID
.
-
The code below shows how to use the license:
FaceAttribute-Flutter/lib/main.dart
Lines 71 to 79 in d5e05cd
-
To request a license, please contact us:
π§Email:
contact@kby-ai.com
π§Telegram:
@kbyai
π§WhatsApp:
+19092802609
π§Skype:
live:.cid.66e2522354b1049b
π§Facebook:
https://www.facebook.com/KBYAI
Make sure you have Flutter installed.
This repo has been built with Flutter version 3.22.3
.
If you don't have Flutter installed, please follow the instructions provided in the official Flutter documentation here.
Run the following commands:
flutter pub upgrade
flutter run
If you plan to run the iOS app, please refer to the following link for detailed instructions.
Android
- Copy the SDK (folder
libfacesdk
) to the folderandroid
in your project. - Add SDK to the project in
settings.gradle
.
include ':libfacesdk'
- Copy the folder
facesdk_plugin
to theroot
folder of your project. - Add the dependency in your
pubspec.yaml
file.
facesdk_plugin:
path: ./facesdk_plugin
- Import the
facesdk_plugin
package.
import 'package:facesdk_plugin/facesdk_plugin.dart';
import 'package:facesdk_plugin/facedetection_interface.dart';
- Activate the
FacesdkPlugin
by calling thesetActivation
method:
final _facesdkPlugin = FacesdkPlugin();
...
await _facesdkPlugin
.setActivation(
"Os8QQO1k4+7MpzJ00bVHLv3UENK8YEB04ohoJsU29wwW1u4fBzrpF6MYoqxpxXw9m5LGd0fKsuiK"
"fETuwulmSR/gzdSndn8M/XrEMXnOtUs1W+XmB1SfKlNUkjUApax82KztTASiMsRyJ635xj8C6oE1"
"gzCe9fN0CT1ysqCQuD3fA66HPZ/Dhpae2GdKIZtZVOK8mXzuWvhnNOPb1lRLg4K1IL95djy0PKTh"
"BNPKNpI6nfDMnzcbpw0612xwHO3YKKvR7B9iqRbalL0jLblDsmnOqV7u1glLvAfSCL7F5G1grwxL"
"Yo1VrNPVGDWA/Qj6Z2tPC0ENQaB4u/vXAS0ipg==")
.then((value) => facepluginState = value ?? -1);
- Initialize the
FacesdkPlugin
:
await _facesdkPlugin
.init()
.then((value) => facepluginState = value ?? -1)
- Set parameters using the
setParam
method:
await _facesdkPlugin.setParam({
'check_liveness_level': livenessLevel ?? 0,
'check_eye_closeness': true,
'check_face_occlusion': true,
'check_mouth_opened': true,
'estimate_age_gender': true
});
- Extract faces using the
extractFaces
method:
final faces = await _facesdkPlugin.extractFaces(image.path)
- Calculate the similarity between faces using the
similarityCalculation
method:
double similarity = await _facesdkPlugin.similarityCalculation(
face['templates'], person.templates) ??
-1;
To build the native camera screen and process face detection, please refer to the lib/facedetectionview.dart file in the repository.
This file contains the necessary code for implementing the camera screen and performing face detection.