-
Notifications
You must be signed in to change notification settings - Fork 0
Writing plugins
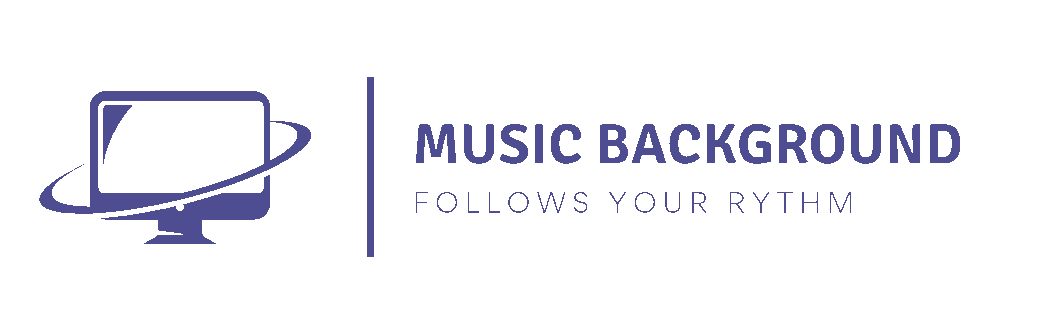
Each plugin is simple python library. Every plugin can add providers of variables and image processors.
Plugins are found by using entrypoints mechanism.
Entrypoint for variable providers is "mbg_variables".
If you use setup.py then add entry_points as showed below:
setup(
name="myproject",
packages=["myproject"],
# the following makes a plugin available to music_bg
entry_points={"mbg_variables": ["variable_name = myproject.pluginmodule:variable_function"]},
)
If you use poetry then add this to your pyproject.toml
[tool.poetry.plugins.mbg_variables]
variable_name = "myproject.pluginmodule:variable_function"
variable provider takes only one argument: current music_bg context. It's an instance of a class music_bg.context.Context.
Example of a valid variable provider:
def uuid4() -> uuid.UUID:
"""
Generate and return UUIDv4.
:return: generated UUID.
"""
return uuid.uuid4()
Now you can use "{uuid4}" in your config file.
Entrypoint for variable providers is "mbg_processors".
If you use setup.py then add entry_points as showed below:
setup(
name="myproject",
packages=["myproject"],
# the following makes a plugin available to music_bg
entry_points={"mbg_processors": ["processor_name = myproject.pluginmodule:processor_function"]},
)
If you use poetry then add this to your pyproject.toml
[tool.poetry.plugins.mbg_processors]
processor_name = "myproject.pluginmodule:processor_function"
Each processor function takes an image (Pillow-SIMD) as a required argument. Optionally you can add additional parameter. These parameters are passed through config file.
Each parameter can be a string, because we need to use them for substituting variables.
Example of a processor_function:
from typing import Union
from PIL.Image import Image
from PIL.ImageFilter import BoxBlur, GaussianBlur
def box_blur(image: Image, strength: Union[str, int] = 5) -> Image:
"""
Apply BoxBlur filter onto an image.
:param image: Input image.
:param strength: Blur strength.
:return: Blurred image.
"""
return image.filter(BoxBlur(int(strength)))
Now you can add this image processor:
{
"name": "box_blur",
"args": {
"strength": "6"
}
}
As an example of a plugin you can use music_bg_extra: https://github.com/music-bg/music_bg_extra/