-
Notifications
You must be signed in to change notification settings - Fork 8.8k
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
optimize: support oracle date types #4542
Changes from 68 commits
dd38eae
1d2bce2
7dde6f4
6c2d330
9c41301
a772160
806f812
edac1e4
143cf18
ff833d1
85b66ec
1429677
c6970e6
75e2b68
02fa91b
3c2c268
ce9886a
0572e16
dbb4376
dcceeff
19bf5a6
db243ec
5c68d2c
bc04c40
2b7edb3
db0326c
ee798d4
348a0a2
c4993fc
4328c07
bf62829
6c87f34
1c7b8f7
82580c7
e6f8194
8e3fa32
f663515
4899fa8
3d9b17c
4baba76
7bba2bb
575c879
e917094
ac85f0e
d6ee786
0dbad8b
08a0347
8ecea26
20e9318
770bb77
3811a43
5dd5f4d
bf319cc
5dac2cc
f35e745
76007c7
e435219
6f35f45
826c4e1
ae59a06
a24f18c
098ddc8
b6184eb
8025cbf
c9fb36c
9e16549
922df89
239c709
b740f8a
757ae77
7291166
File filter
Filter by extension
Conversations
Jump to
Diff view
Diff view
There are no files selected for viewing
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,26 @@ | ||
/* | ||
* Copyright 1999-2019 Seata.io Group. | ||
* | ||
* Licensed under the Apache License, Version 2.0 (the "License"); | ||
* you may not use this file except in compliance with the License. | ||
* You may obtain a copy of the License at | ||
* | ||
* http://www.apache.org/licenses/LICENSE-2.0 | ||
* | ||
* Unless required by applicable law or agreed to in writing, software | ||
* distributed under the License is distributed on an "AS IS" BASIS, | ||
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. | ||
* See the License for the specific language governing permissions and | ||
* limitations under the License. | ||
*/ | ||
package io.seata.rm.datasource.exec.oracle; | ||
|
||
/** | ||
* @author doubleDimple lovele.cn@gmail.com | ||
*/ | ||
public class OracleJdbcType { | ||
|
||
public static final int TIMESTAMP_WITH_TIME_ZONE = -101; | ||
|
||
public static final int TIMESTAMP_WITH_LOCAL_TIME_ZONE = -102; | ||
} |
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,115 @@ | ||
/* | ||
* Copyright 1999-2019 Seata.io Group. | ||
* | ||
* Licensed under the Apache License, Version 2.0 (the "License"); | ||
* you may not use this file except in compliance with the License. | ||
* You may obtain a copy of the License at | ||
* | ||
* http://www.apache.org/licenses/LICENSE-2.0 | ||
* | ||
* Unless required by applicable law or agreed to in writing, software | ||
* distributed under the License is distributed on an "AS IS" BASIS, | ||
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. | ||
* See the License for the specific language governing permissions and | ||
* limitations under the License. | ||
*/ | ||
package io.seata.rm.datasource.util; | ||
|
||
import java.time.LocalDateTime; | ||
import java.time.OffsetDateTime; | ||
import java.time.ZoneId; | ||
import java.time.ZoneOffset; | ||
import java.time.format.DateTimeFormatter; | ||
import java.util.HashMap; | ||
import java.util.Map; | ||
|
||
import static java.lang.Short.toUnsignedInt; | ||
import static java.time.ZoneOffset.UTC; | ||
|
||
/** | ||
* @author doubleDimple | ||
*/ | ||
public class OffsetTimeUtils { | ||
|
||
public static final String PATTERN_1 = "yyyy-MM-dd HH:mm:ss.SSS"; | ||
|
||
public static final String PATTERN_2 = "yyyy-MM-dd HH:mm:ss"; | ||
|
||
private static final byte REGIONIDBIT = (byte)0b1000_0000; | ||
|
||
private static Map<Integer, String> ZONE_ID_MAP = new HashMap<>(2); | ||
|
||
public static String getRegion(int code) { | ||
return ZONE_ID_MAP.get(code); | ||
} | ||
|
||
public static String convertOffSetTime(OffsetDateTime offsetDateTime) { | ||
if (null != offsetDateTime) { | ||
return offsetDateTime.format(DateTimeFormatter.ofPattern(PATTERN_1)); | ||
} | ||
return null; | ||
} | ||
|
||
public static OffsetDateTime timeToOffsetDateTime(byte[] bytes) { | ||
if (null == bytes || bytes.length == 0) { | ||
return null; | ||
} | ||
|
||
OffsetDateTime utc = extractUtc(bytes); | ||
if (bytes.length >= 8) { | ||
if (isFixedOffset(bytes)) { | ||
ZoneOffset offset = extractOffset(bytes); | ||
return utc.withOffsetSameInstant(offset); | ||
} else { | ||
ZoneId zoneId = extractZoneId(bytes); | ||
return utc.atZoneSameInstant(zoneId).toOffsetDateTime(); | ||
} | ||
} | ||
return utc.atZoneSameInstant(ZoneId.systemDefault()).toOffsetDateTime(); | ||
} | ||
|
||
private static boolean isFixedOffset(byte[] bytes) { | ||
return (bytes[11] & REGIONIDBIT) == 0; | ||
} | ||
|
||
private static OffsetDateTime extractUtc(byte[] bytes) { | ||
return OffsetDateTime.of(extractLocalDateTime(bytes), UTC); | ||
} | ||
|
||
private static ZoneId extractZoneId(byte[] bytes) { | ||
// high order bits | ||
int regionCode = (bytes[11] & 0b1111111) << 6; | ||
// low order bits | ||
regionCode += (bytes[12] & 0b11111100) >> 2; | ||
String regionName = getRegion(regionCode); | ||
return ZoneId.of(regionName); | ||
} | ||
|
||
private static ZoneOffset extractOffset(byte[] bytes) { | ||
int hours = bytes[11] - 20; | ||
int minutes = bytes[12] - 60; | ||
if ((hours == 0) && (minutes == 0)) { | ||
return ZoneOffset.UTC; | ||
} | ||
return ZoneOffset.ofHoursMinutes(hours, minutes); | ||
} | ||
|
||
private static LocalDateTime extractLocalDateTime(byte[] bytes) { | ||
int year = ((toUnsignedInt(bytes[0]) - 100) * 100) + (toUnsignedInt(bytes[1]) - 100); | ||
int month = bytes[2]; | ||
int dayOfMonth = bytes[3]; | ||
int hour = bytes[4] - 1; | ||
int minute = bytes[5] - 1; | ||
int second = bytes[6] - 1; | ||
int nanoOfSecond = 0; | ||
if (bytes.length >= 8) { | ||
nanoOfSecond = (toUnsignedInt(bytes[7]) << 24) | (toUnsignedInt(bytes[8]) << 16) | ||
| (toUnsignedInt(bytes[9]) << 8) | toUnsignedInt(bytes[10]); | ||
} | ||
return LocalDateTime.of(year, month, dayOfMonth, hour, minute, second, nanoOfSecond); | ||
} | ||
|
||
static { | ||
ZONE_ID_MAP.put(250, "Asia/Shanghai"); | ||
There was a problem hiding this comment. Choose a reason for hiding this commentThe reason will be displayed to describe this comment to others. Learn more. how to ensure all the There was a problem hiding this comment. Choose a reason for hiding this commentThe reason will be displayed to describe this comment to others. Learn more. 具体解决办法:1维护常用的时区,其他时区通过在配置文件中配置,实时读取相关配置 There was a problem hiding this comment. Choose a reason for hiding this commentThe reason will be displayed to describe this comment to others. Learn more.
We first add the commonly used time zones, the remaining open interface allows users to manually add their own, 1. api way to add time zones 2. configuration file to add time zones , both options should be supported There was a problem hiding this comment. Choose a reason for hiding this commentThe reason will be displayed to describe this comment to others. Learn more. |
||
} | ||
} |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Be careful not to duplicate the int value of java.sql.Types.
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
The following are duplicate types:
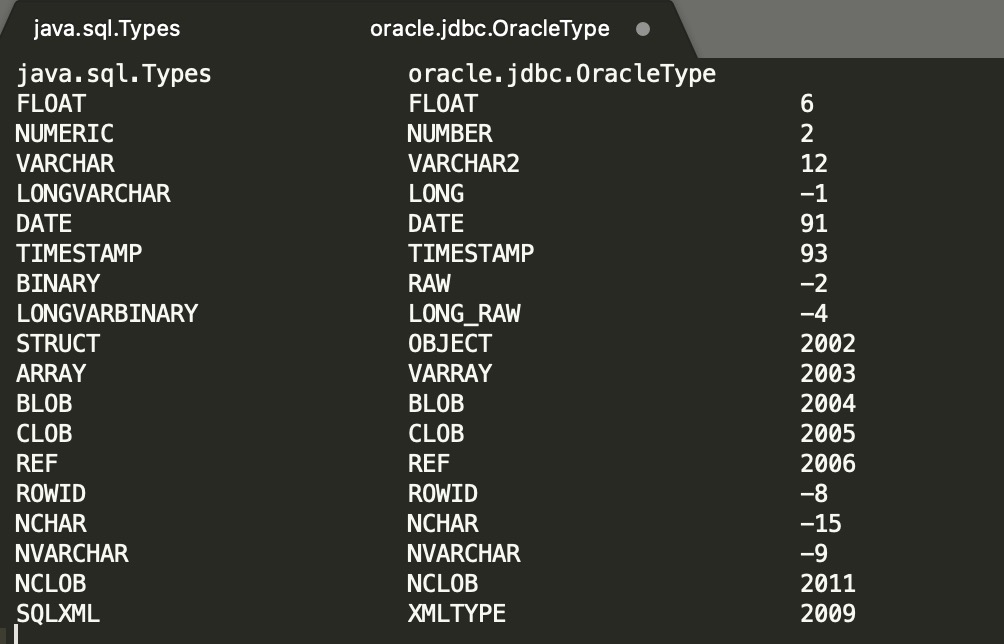